Get Session Token
You must create a session token to interact with Amberflo through: -API -UI Kit -AmberfloSDK.
To do so, you can retrieve it from an endpoint on your server using the browser’s fetch function on the client side. This is generally the best approach when your client side is a single page application, especially if it’s built with a modern frontend framework such as React.
For more information check API Reference
This example shows how to create the server endpoint that serves the client secret:
📘 The above example is in Node.js
You can get X-API-KEY from Amberflo site inside Settings -> Account -> API keys.
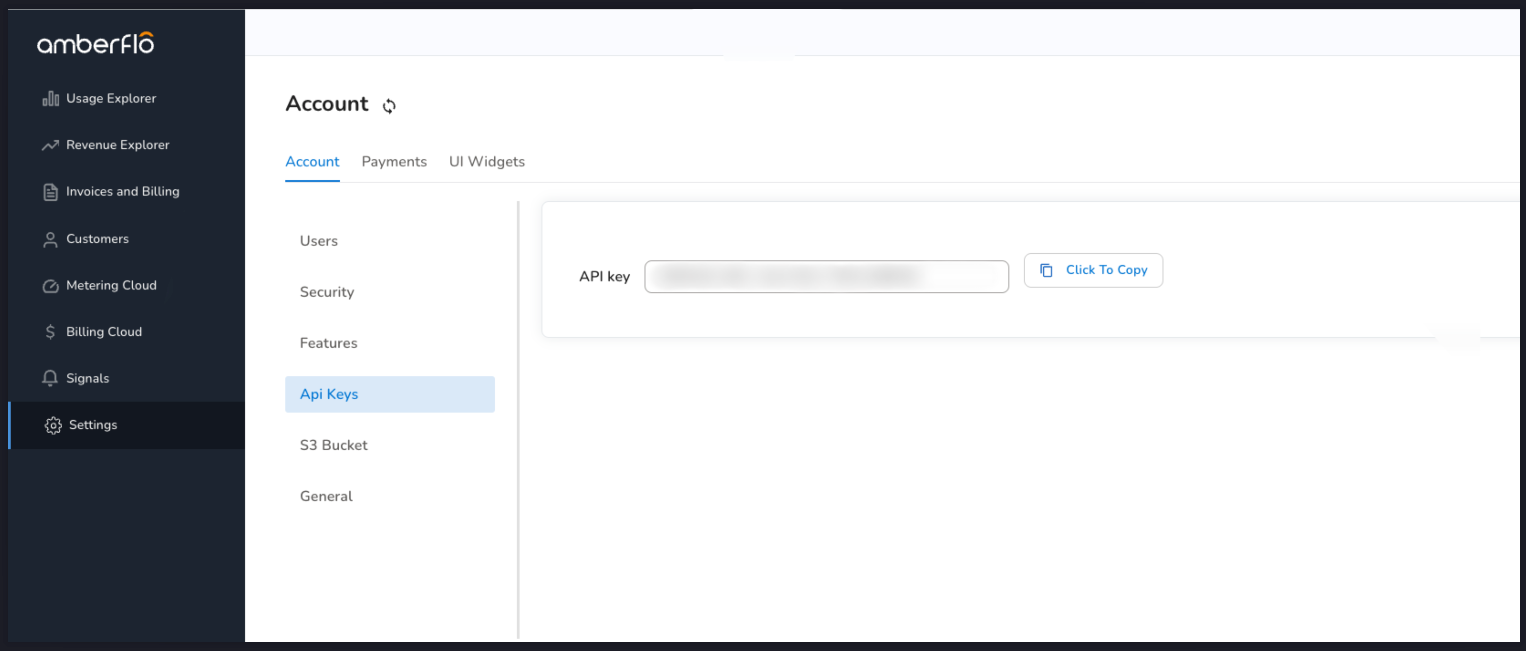
You can also get the customerId from Amberflo site inside Customers → Click on a customer → Copy Customer ID.
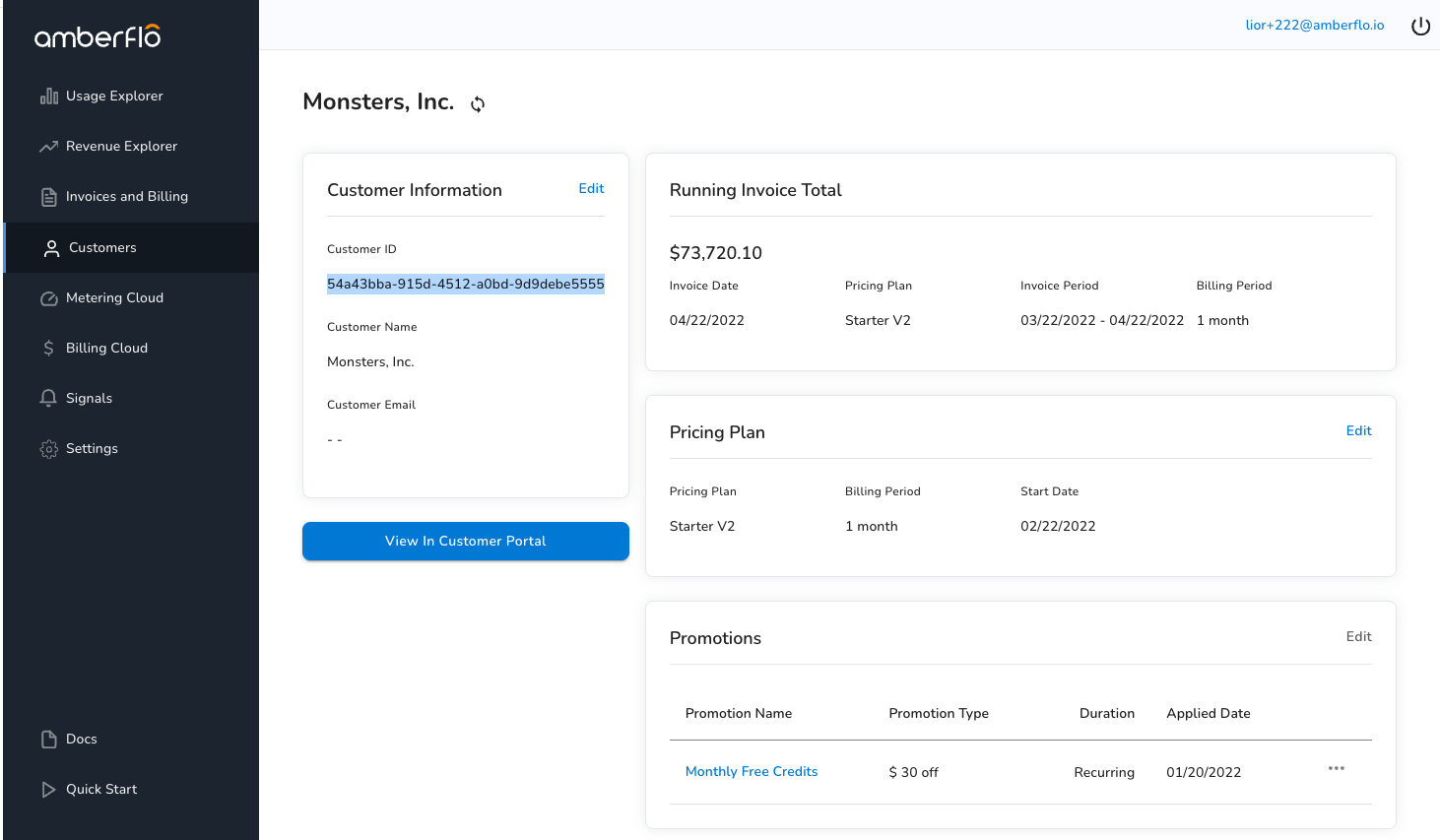
This example demonstrate how to fetch the session token with JavaScript in the client side: